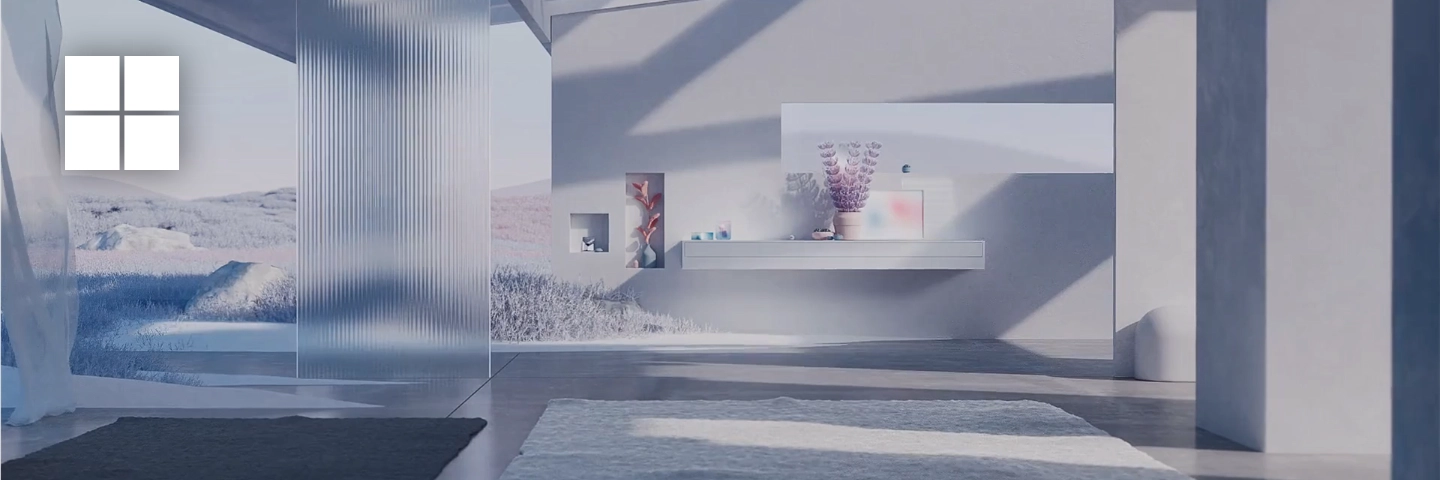
WPF 开发经验笔记
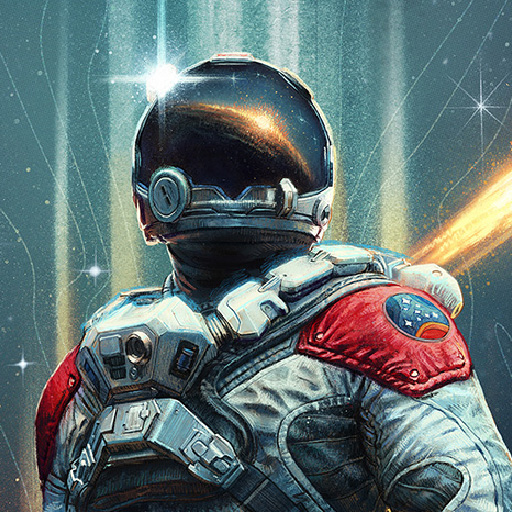
记录一些可能用上的开发技巧与常用代码,方便之后查阅。
启动窗口
更改 App.xaml
文件,StartupUri
为启动窗口。
1 | <Application ... |
异形窗体和亚克力特效(WindowChrome 相关)
参考文章:
- 使用 SetWindowCompositionAttribute 来控制程序的窗口边框和背景(可以做 Acrylic 亚克力效果、模糊效果、主题色效果等)
- WPF 制作高性能的透明背景异形窗口(使用 WindowChrome 而不要使用 AllowsTransparency=True)
如何接管窗体关闭按钮逻辑并实现最小化到托盘
使用开源控件库 Handy Control 实现托盘图标。
1 | private void Window_Closing(object sender, System.ComponentModel.CancelEventArgs e) |
实现单例模式
1 | private static Base64Tool instance; |
async/await 关键字实现异步操作
1 | public async void Screenshot_Snapped(string str) |
WPF 资源使用
分为二进制资源和逻辑资源。二进制资源主要为图片等资源,逻辑资源如 Xaml 各个元素的 Resource 属性,资源字典 ResourceDictionary.
二进制资源 Resource 嵌入
资源会被嵌入最终生成的 exe 或 dll 中,在 WPF 中可以通过 URI 访问。
- 设置资源为 Resource
- 把资源加入工程
- 设置资源属性 “生产操作” -> “Resource”
- 访问方式(URI)
<Image Source="pack://application:,,,/Learn.Library;component/s.gif" />
<Image Source="/Learn.Library;component/s.gif"/>
<Image Source="/ResourceDll;component/Resources/cancel.png"/>
myBitmapImage.UriSource = new Uri(“pack://application:,/ResourceDll;component/Resources/cancel.png”, UriKind.Absolute);
二进制资源 Loose File
资源不会被嵌入,而是运行时加载,方便用户切换。然而当资源丢失或无法被正常打开时,会导致程序崩溃。
- 调用方式
<Image Source="pack://siteoforigin:,,,/Images/Cancel.png"/>
myBitmapImage.UriSource = new Uri(“pack://siteoforigin:,/Images/Cancel.png”, UriKind.Absolute);
<Image Source="C:\Images/Cancel.png"/>
<Image Source="file://C:Images/Cancel.png"/>
<Image Source="http://www.qidian.com/images/logo.gif" />
<Image Source="\\server1\share\logo.gif" />
- 标题: WPF 开发经验笔记
- 作者: Zahkrii
- 创建于 : 2022/03/12 11:23:22
- 更新于 : 2024/06/24 23:30:56
- 链接: https://zahkrii.github.io/2022/03/12/wpf-notes/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。